目录
介绍
- Global Positioning System(GPS)利用了通过在地球上的空间和地面站卫星发送来准确地确定其在地球上的位置的信号。
- 从卫星和地面站发送的射频信号由GPS接收。GPS利用这些信号确定其准确位置。
- GPS本身不需要传输任何信息。
- 从卫星和地面站接收的信号包含信号传输时的时间戳。通过计算信号传输时间和信号接收时间之间的差异。使用信号的速度,可以使用速度和时间的距离的简单公式来确定卫星和GPS接收器之间的距离。
- 使用来自3颗或更多颗卫星的信息,可以对GPS的确切位置进行三角测量。
- GPS接收器模块使用UART通信与控制器或PC终端通信。
- 在Raspberry Pi上使用UART之前,我们应该配置并启用它。
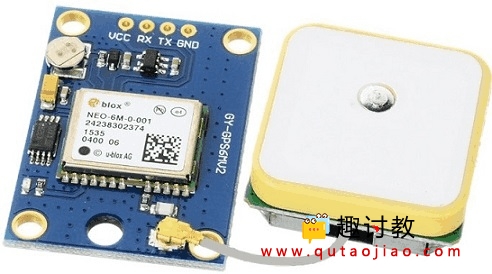
GPS模块
电路连接图
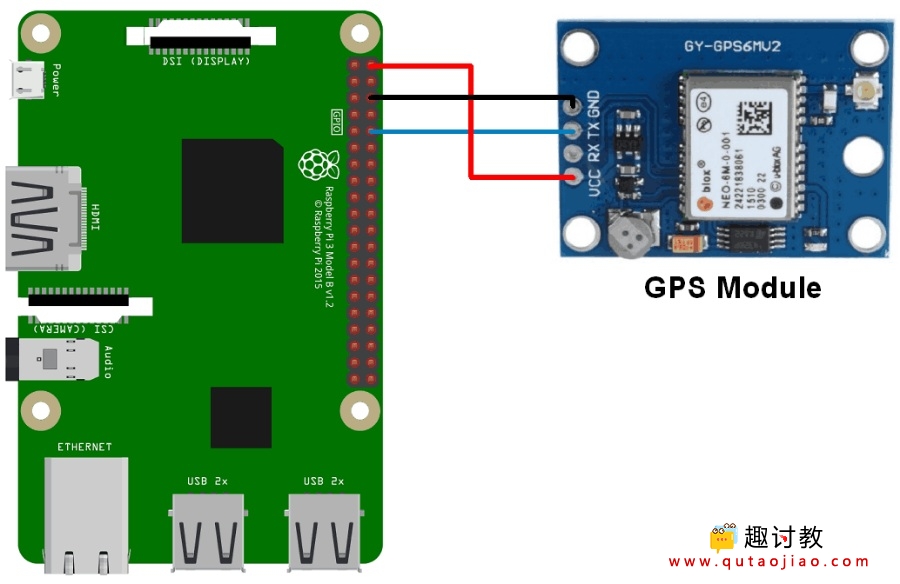
GPS模块与Raspberry Pi连接
例
让我们用Raspberry Pi连接GPS模块,并提取GPS信息。我们可以使用Python和C(WiringPi)将GPS模块连接到Raspberry Pi。要连接GPS模块,请将GPS模块连接到Raspberry Pi,如上图所示。
使用Python语法
让我们使用Python从GPS模块接收的NMEA GPGGA字符串中提取纬度,经度和时间信息。并在控制台(终端)上打印它们。通过使用这些纬度和经度,在Google地图上找到当前位置。
提示:Google地图在国内不需要科学上网也可以进行访问。
Python程序
'''
GPS Interfacing with Raspberry Pi using Pyhton
https://www.qutaojiao.com
'''
import serial #import serial pacakge
from time import sleep
import webbrowser #import package for opening link in browser
import sys #import system package
def GPS_Info():global NMEA_buff
global lat_in_degrees
global long_in_degrees
nmea_time = []
nmea_latitude = []
nmea_longitude = []
nmea_time = NMEA_buff[0] #extract time from GPGGA string
nmea_latitude = NMEA_buff[1] #extract latitude from GPGGA string
nmea_longitude = NMEA_buff[3] #extract longitude from GPGGA string
print("NMEA Time: ", nmea_time,'n')
print ("NMEA Latitude:", nmea_latitude,"NMEA Longitude:", nmea_longitude,'n')
lat = float(nmea_latitude) #convert string into float for calculation
longi = float(nmea_longitude) #convertr string into float for calculation
lat_in_degrees = convert_to_degrees(lat) #get latitude in degree decimal format
long_in_degrees = convert_to_degrees(longi) #get longitude in degree decimal format
#convert raw NMEA string into degree decimal format
def convert_to_degrees(raw_value):
decimal_value = raw_value/100.00
degrees = int(decimal_value)
mm_mmmm = (decimal_value - int(decimal_value))/0.6
position = degrees + mm_mmmm
position = "%.4f" %(position)
return position
gpgga_info = "$GPGGA,"
ser = serial.Serial ("/dev/ttyS0") #Open port with baud rate
GPGGA_buffer = 0
NMEA_buff = 0
lat_in_degrees = 0
long_in_degrees = 0
try:
while True:
received_data = (str)(ser.readline()) #read NMEA string received
GPGGA_data_available = received_data.find(gpgga_info) #check for NMEA GPGGA string if (GPGGA_data_available>0):
GPGGA_buffer = received_data.split("$GPGGA,",1)[1] #store data coming after "$GPGGA," string
NMEA_buff = (GPGGA_buffer.split(',')) #store comma separated data in buffer
GPS_Info() #get time, latitude, longitude
print("lat in degrees:", lat_in_degrees," long in degree: ", long_in_degrees, 'n')
map_link = 'http://maps.google.com/?q=' + lat_in_degrees + ',' + long_in_degrees #create link to plot location on Google map
print("<<<<<<<<press ctrl+c to plot location on google maps>>>>>>n") #press ctrl+c to plot on map and exit
print("------------------------------------------------------------n")
余下程序(直接放于上面程序的下面):
Python的输出
- Python IDE上的输出
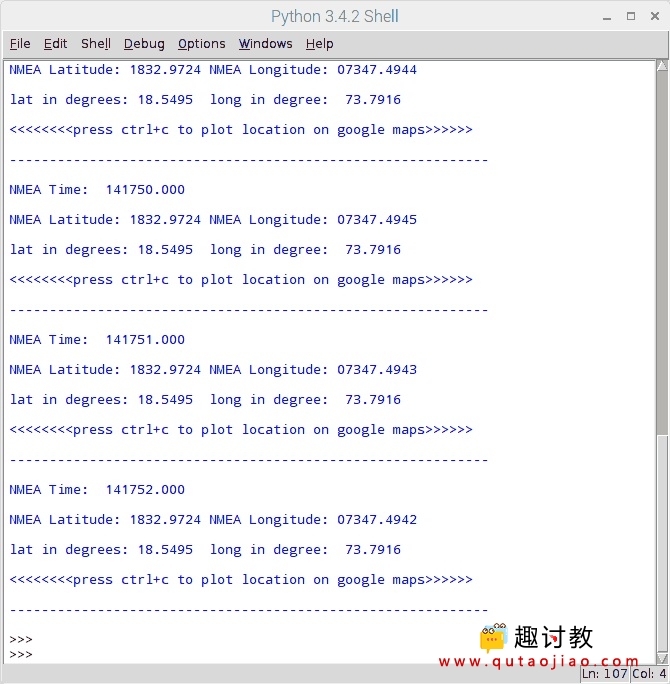
输出窗口
- Google地图上的输出位置
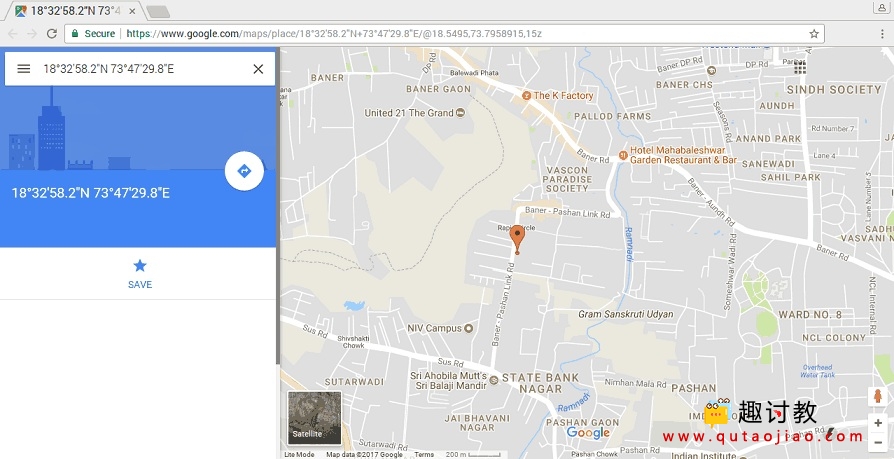
谷歌地图上的位置
要在Google地图上绘制我们的位置,我们需要调用Google地图的网址链接。我们可以使用以下链接打开谷歌地图与我们提取的经度和纬度。
http://maps.google.com/?q=<latitude>,<longitude>
使用C语法
我们将提取NMEA GPGGA字符串并将其打印在输出窗口中。在这里,我们使用C语言编写的WiringPi库来读取GPS模块。
C程序
/*
GPS Interfacing with Raspberry Pi using C (WiringPi Library)
https://www.qutaojiao.com
*/
#include <stdio.h>
#include <string.h>
#include <errno.h>
#include <wiringPi.h>
#include <wiringSerial.h>
int main ()
{
int serial_port;
char dat,buff[100],GGA_code[3];
unsigned char IsitGGAstring=0;
unsigned char GGA_index=0;
unsigned char is_GGA_received_completely = 0;if ((serial_port = serialOpen ("/dev/ttyS0", 9600)) < 0) /* open serial port */
{
fprintf (stderr, "Unable to open serial device: %sn", strerror (errno)) ;
return 1 ;
}
if (wiringPiSetup () == -1) /* initializes wiringPi setup */
{
fprintf (stdout, "Unable to start wiringPi: %sn", strerror (errno)) ;
return 1 ;
}
while(1){
if(serialDataAvail (serial_port) ) /* check for any data available on serial port */
{
dat = serialGetchar(serial_port); /* receive character serially */
if(dat == '$'){
IsitGGAstring = 0;
GGA_index = 0;
}
else if(IsitGGAstring ==1){
buff[GGA_index++] = dat;
if(dat=='r')
is_GGA_received_completely = 1;
}
else if(GGA_code[0]=='G' && GGA_code[1]=='G' && GGA_code[2]=='A'){
IsitGGAstring = 1;
GGA_code[0]= 0;
GGA_code[0]= 0;
GGA_code[0]= 0;
}
else{
GGA_code[0] = GGA_code[1];
GGA_code[1] = GGA_code[2];
GGA_code[2] = dat;
}
}
余下程序(直接拼接到上面程序之下即可):
输出
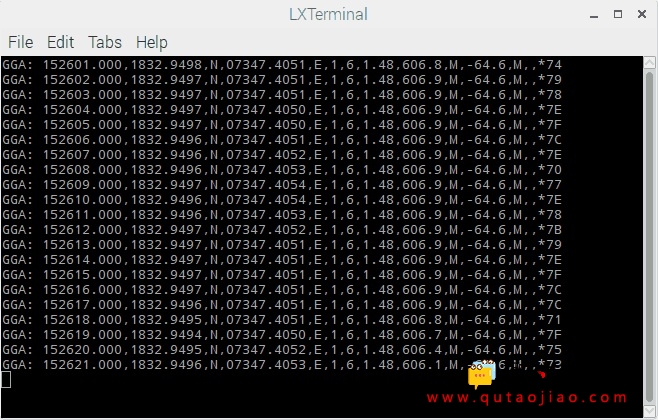
C程序的输出
注意:当我们使用Raspberry Pi 3连接GPS模块时,我们使用/dev/ttyS0串口进行UART通信。那些与Raspberry Pi 2和之前的型号接口的人,你应该使用/dev/ttyAMA0代替。
请问串口获得的原始数据是什么样的